Under the API Access Control tab, you can configure the following options:
Authentication – The three different types of authentication options available are API keys, Basic Authentication and JWT tokens.
Users – Manage which specific users (or API consumers) have access to the API.
Groups – Assign access permissions to specific user groups.
IP Addresses – Restrict access to the API by specifying allowed IP addresses.
Silverline API supports two types of users, also called API consumers, as they may include both physical users and service accounts.
Internal API Consumers – These are user accounts or service accounts created and managed inside Silverline API application, either manually or through the User API.
External API Consumers – These are user accounts or service accounts with credentials stored outside Silverline API, like in a database table or a flat file (CSV or Excel). Silverline API manages their authentication independently, without relying on an external system. It also supports integration with Microsoft Active Directory.
AUTHENTICATION –
Navigate to: APIs > Edit API ( or Create API)
[Note: For new APIs the API Access Control tab becomes visible only after saving the query or script for the API data.]
Under the Authentication section in the API Access Control tab, you can choose from the following authentication methods: JWT, Basic Auth and API Key
JWT (JSON Web Token)
– For Internal API Consumers
(See below for External API Consumers)
To obtain your JWT token, follow these steps:
Access the Login API:
Navigate to the Login API endpoint (first API on the APIs tab – named as ‘Login API’), which is used for authentication of internal consumers.
Provide Credentials:
Send a POST request to the Login API with your user ID and password as the request body.
If the provided credentials are valid, the server will respond with a JWT token in the response body.
{
“token”: “bearer xxxxxxxx”,
}
3. Save the JWT token as it will be required for authenticating subsequent API requests.
4. Include the token in the Authorization header of your API requests using the Bearer keyword.
Example of using JWT token in a request header:
Authorization: Bearer <your-JWT-token>

Note: To implement JWT Bearer tokens for internal API consumers, ensure that any API using this authentication method has the necessary users (API consumers) or groups set up for access. This is setup under the API Access controls tab > Users or Groups
Sample Javascript code snippet for JWT Bearer auth for internal API consumers –
In this example, we are accessing the Payments Due API, which is authenticated using JWT tokens.
// Step 1: Get JWT token from Login API
fetch(“https://silverlineapi.com/app/api/v1/login”, {
method: “POST”,
headers: { “Content-Type”: “application/json” },
body: JSON.stringify({ email: “user1@example.com”, password: “password1” })
})
.then(response => response.json())
.then(data => {
const jwtToken = data.token; // Extract JWT token
// Step 2: Use JWT token to call Due Payments API
return fetch(“https://silverlineapi.com/app/api/v1/duePayments”, {
method: “POST”,
headers: {
“Authorization”: `Bearer ${jwtToken}`,
“Content-Type”: “application/json”
}
});
})
.then(response => response.json())
.then(data => console.log(“API Response:”, data))
.catch(error => console.error(“Error:”, error));
Basic Authentication – For Internal API Consumers
(See below for External API Consumers)
Basic authentication is a simple method where the username and password is sent with every API request, typically encoded as base64.

In your script or HTTP request, include the Authorization header in this format:
Authorization: Basic dXNlcjE7MzpreLN0Y3NyZVBsc3N3b3Jk
Where dXNlcjE7MzpreLN0Y3NyZVBsc3N3b3Jk is the base64 encoded value of User Name and Password.
Note: To implement JWT Bearer tokens for internal API consumers, ensure that any API using this authentication method has the necessary users (API consumers) or groups set up for access. This is setup under the API Access controls tab > Users or Groups
Sample Javascript code snippet for Basic auth for internal API consumers-
// API endpoint
const apiUrl = “https://silverlineapi.com/app/api/v1/duePayments”;
// User credentials for Basic Authentication
const email = “user1@example.com”;
const password = “password1”;
// Encode credentials in Base64
const authHeader = “Basic ” + btoa(`${username}:${password}`);
// Make the API request with Basic Auth
fetch(apiUrl, {
method: “POST”,
headers: {
“Authorization”: authHeader, // Include Basic Auth header
“Content-Type”: “application/json”
}
})
.then(res => res.json()) // Parse JSON response
.then(data => console.log(“API Response:”, data)) // Log API response
.catch(err => console.error(“Error:”, err)); // Handle errors
JWT (JSON Web Token) – For External API Consumers
(See above for Internal API Consumers)
To authenticate an external API consumers, start by creating a Login API on the data source where the external API consumers are stored. This Login API will validate the user’s credentials and return a signed JWT token.
If authentication is successful, the token will include the API consumer’s assigned groups in the JWT’s claims, which will be used for authorization in subsequent API calls. If authentication fails, the token will not contain any group information. To access protected resources, include the JWT token in each API call for authentication and authorization.
To obtain your JWT token, follow these steps –
Create a Login API:
Navigate to – APIs tab and click on New API button.
Select your data source and check the box labeled “Are you creating a Login API?”. (Note that selecting this option ensures the Silverline API application responds with a signed JWT Bearer token.)
This API will be used to authenticate users (API consumers) by validating their username and password and will return a signed JWT token.
The token will include the user’s (API consumer’s) assigned groups in the x-silverlineapi-groups variable as a JSON array.
Important Note:
External API consumers must be assigned at least one group to authenticate successfully. This group is essential for authorizing their API requests.
The x-silverline-groups variable should be included in the Login API response as a string or a JSON array, specifying the user’s (API consumer’s) assigned groups. If no group is assigned, authentication will fail.
If an API consumers is assigned to multiple groups, ensure they are formatted as a JSON array
Here is an example of authenticating external API consumers :
In this example, external users (API consumers) are stored in a database called Car Dealership, within the Finance_Team_Members table. These users belong to a group called Finance_Team, which has exclusive access to certain company financial APIs.
To begin, we will create a Login API to authenticate these users (API consumers).
It is important to first ensure that all finance-related APIs are properly configured to restrict access to the Finance_Team group. Refer to the “Users and Groups“ documentation for details on how to create and enforce group level restrictions within Silverline API.
Connect the Car Dealership database to Silverline API application by navigating to the Data Sources tab. Once connected and all the necessary tables are selected save the configuration.
Now navigate to the APIs tab and click on the New API button. Select the Car dealership database from the drop down and Check the box “Are you creating a Login API?”
Enter an API Name – “Car Dealership Login API”
Type in natural language (or you can manually write the query too):
Construct a query to validate {{username}} and {{password}} against the Finance_Team_Members table. The password should be hashed using SHA2 for comparison. If a match is found, return the user details and include an attribute x-silverline-groups with the value Finance_Team.
[Tip: If you encounter errors while using natural language, simply copy the error message into the Question box. In most cases, AI will resolve it for you.]
Silverline API automatically builds the query with the required parameters. You can test these parameters, and once satisfied with the results, save and publish the query.
Sample PostgreSQL query SIlverline API generated –
SELECT
username AS “Username”,
‘Finance_Team’ AS “x-silverline-groups”
FROM
Finance_Team_Members
WHERE
username = ‘{{username}}’
AND password_hash = encode(digest(‘{{password}}’, ‘sha256’), ‘hex’);
[Tip: If a user (API consumer) is assigned to multiple groups, ensure they are formatted as a JSON array]
Next, validate the query using the API Endpoint tab. (Note: The API Endpoint becomes available only after saving the previous step.)
For testing, enter a sample username and password as shown in the example below. You should receive a JWT Bearer token, which can be used for subsequent API calls.
Note: You can assign a friendly name to your APIs, as shown in this example where “login_v1” was set through the Optional Settings tab.
Note : Your Login API is available under the API Endpoint tab.
Using the JWT Token
Let’s say we want to access the “List Customers with Due Payments” API.
First, we need to confirm whether the Finance_Team group is permitted to access it. To do this, navigate to the APIs tab, select the “List Customers with Due Payments” API, and click the Edit (pen) icon to review its access settings.
Now navigate to API Access Control > Groups
From the image above, we can confirm that Finance_Team is the only group allowed to access this API.
Then make sure under the Authentication Tab – JWT Bearer is selected as the authentication method.
Next, send the JWT token generated from the “Car Dealership Login API” to the “List Customers with Due Payments” API endpoint, in the Authorization header.
Sample Javascript code snippet for JWT Bearer auth for external API consumers –
// Step 1: Get JWT token from Login API
fetch(“https://silverlineapi.com/app/api/v1/login_v1?int_key=ef08120b”, {
method: “POST”,
headers: { “Content-Type”: “application/json” },
body: JSON.stringify({ username: “user1”, password: “password1” })
})
.then(response => response.json())
.then(data => {
const jwtToken = data.token; // Extract JWT token
// Step 2: Use JWT token to call Due Payments API
return fetch(“https://silverlineapi.com/app/api/v1/duePayments”, {
method: “POST”,
headers: {
“Authorization”: `Bearer ${jwtToken}`,
“Content-Type”: “application/json”
}
});
})
.then(response => response.json())
.then(data => console.log(“API Response:”, data))
.catch(error => console.error(“Error:”, error));
Basic Authentication – For External API Consumers
(See above for Internal API Consumers)
The Basic Authentication process for external API consumers consists of two steps.
First, create a Login API that validates API consumers credentials. Upon successful authentication, the API returns the group(s) the API consumer belongs to in the x-silverline-groups variable.
In the second step, any API requiring Basic Authentication for external API consumers must be linked to the Login API. This ensures that the username and password are sent for validation before granting access to the requested API.
Creating a Login API :
Navigate to – APIs tab and click on New API button.
Select your data source which has the external API consumers and check the box labeled “Are you creating a Login API?”. (Note: Selecting this option ensures that the Silverline API application recognizes this as a Login API, which will be required for mapping later.)
This API will be used to authenticate users (API consumers) by validating their username and password.
The response should include the user’s (API consumer’s) assigned groups in the x-silverlineapi-groups variable, either as a string or a JSON array.
Important Note:
External API consumers must be assigned at least one group to authenticate successfully. This group is essential for authorizing their API requests.
The x-silverline-groups variable should be included in the Login API response as a string or a JSON array, specifying the user’s (API consumer’s) assigned groups. If no group is assigned, authentication will fail.
If a user (API consumer) is assigned to multiple groups, ensure they are formatted as a JSON array.
Here is an example of authenticating external API consumers:
In this example, external API consumers are stored in a database called Car Dealership, within the Finance_Team_Members table (the user name is stored in a column called username and password is stored in a column called password with the value being SHA2 hash). These users belong to a group called Finance_Team, which has exclusive access to certain company financial APIs.
To begin, we will create a Login API to authenticate these users (API consumers).
It is important to first ensure that all finance-related APIs are properly configured to restrict access to the Finance_Team group. Refer to the “Users and Groups“ documentation for details on how to create and enforce group level restrictions within Silverline API.
Connect the Car Dealership database to Silverline API application by navigating to the Data Sources tab. Once connected and all the necessary tables are selected save the configuration.
Now navigate to the APIs tab and click on the New API button. Select the Car dealership database from the drop down and Check the box “Are you creating a Login API?”
Enter an API Name – “Car Dealership Login API”
Type in natural language (or you can manually write the query too):
Construct a query to validate {{username}} and {{password}} against the Finance_Team_Members table. The password should be hashed using SHA2 for comparison. If a match is found, return the user details and include an attribute x-silverline-groups with the value Finance_Team.
[Tip: If you encounter errors while using natural language, simply copy the error message into the Question box. In most cases, AI will resolve it for you.]
Silverline API automatically builds the query with the required parameters. You can test these parameters, and once satisfied with the results, save and publish the query.
Sample query SIlverline API generated-
SELECT
username AS “Username”,
‘Finance_Team’ AS “x-silverline-groups”
FROM
Finance_Team_Members
WHERE
username = ‘{{username}}’
AND password_hash = encode(digest(‘{{password}}’, ‘sha256’), ‘hex’);
[Tip: If a user is assigned to multiple groups, ensure they are formatted as a JSON array]
Next, validate the query using the API Endpoint tab. (Note: The API Endpoint becomes available only after saving the previous step.)
For testing, enter a sample username and password as shown in the example below. You should receive a JWT Bearer token, which is typically used for JWT authentication. However, since we are using Basic Authentication, the response can be ignored.
Note: You can assign a friendly name to your APIs, as shown in this example where “login_v1” was set through the Optional Settings tab.
Note : Your Login API is available under the API Endpoint tab.
Linking Login APIs with any API requiring Basic Auth with external API consumers :
Let’s say we want to access the “List Customers with Due Payments” API.
First, we need to confirm whether the Finance_Team group is permitted to access it. To do this, navigate to the APIs tab, select the “List Customers with Due Payments” API, and click the Edit (pen) icon to review its access settings.
Now navigate to API Access Control > Groups
From the image above, we can confirm that Finance_Team is the only group allowed to access this API.
Then, make sure under the Authentication Tab – Basic Auth is selected as the authentication method.
Since we are using Basic Authentication for external API consumers, select the “Requires External user authentication” checkbox. A dropdown will appear—select the Login API created in the previous step.
Now, map the mandatory fields for Basic Authentication—Username and Password—to the corresponding parameters from the Car Dealership Login API. Simply click the dropdown, and Silverline API will automatically display the available parameters from the Car Dealership Login API for selection.
In this example, the Car Dealership Login API has a POST parameter named “username”, which is mapped to Basic Auth’s Username field.
Similarly, the “password” parameter is mapped to Basic Auth’s Password field.
Sample Javascript code snippet for Basic Auth for external API consumers-
// API endpoint
const apiUrl = “https://silverlineapi.com/app/api/v1/duePayments”;
// User credentials for Basic Authentication
const username = “user1”;
const password = “password1”;
// Encode credentials in Base64
const authHeader = “Basic ” + btoa(`${username}:${password}`);
// Make the API request with Basic Auth
fetch(apiUrl, {
method: “POST”,
headers: {
“Authorization”: authHeader, // Include Basic Auth header
“Content-Type”: “application/json”
}
})
.then(res => res.json()) // Parse JSON response
.then(data => console.log(“API Response:”, data)) // Log API response
.catch(err => console.error(“Error:”, err)); // Handle errors
API Key
The key is included in the API request, as a query string parameter.
The int_key (variable) is used to reference an API key. This key is passed along with API requests to authenticate and authorize access to endpoints.
The server checks the key to authenticate the request.
USERS –
Navigate to: APIs > Edit or Create New API > API Access Control > Users
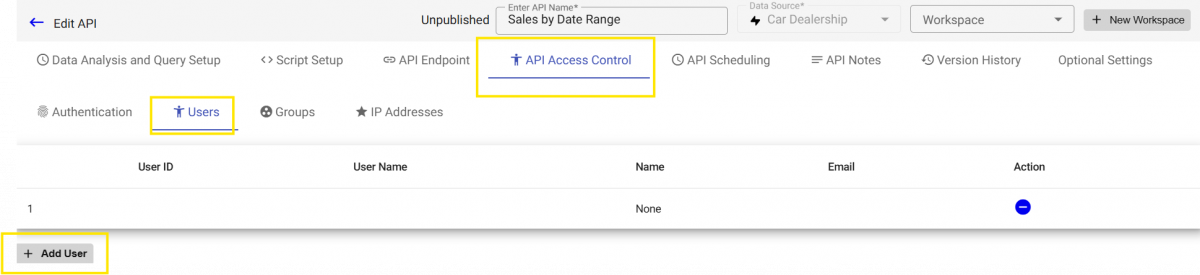
Click the Add User button to grant access to this API. (Users must be created in the Users and Groups navigation tab beforehand.)
Here is the screen shot for Users & Groups for reference. (It’s located under the main navigation header):

GROUPS –
Similar to users, you can restrict API access by allowing only specific groups to access it.
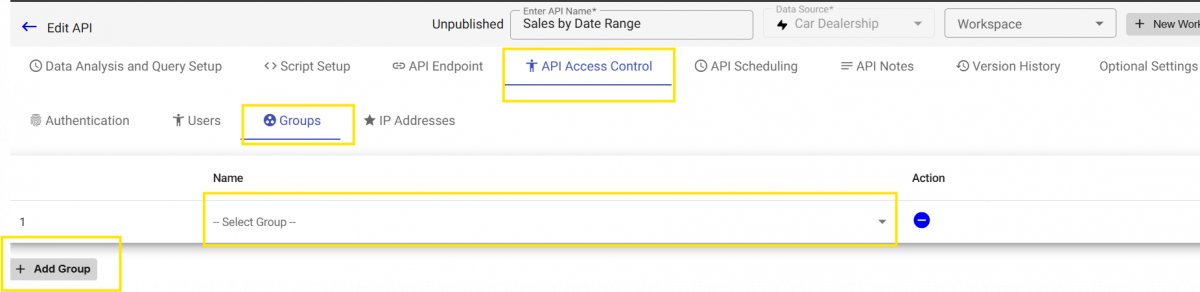
Click the Add Group button to grant access to this API. (Groups must be created in the Users and Groups navigation tab beforehand.)
Here is the screen shot for Users & Groups for reference. (It’s located under the main navigation header):

IP ADDRESS –
Navigate to: APIs > Edit or Create New API > API Access Control > IP Addresses
APIs can also be restricted based on IP addresses.
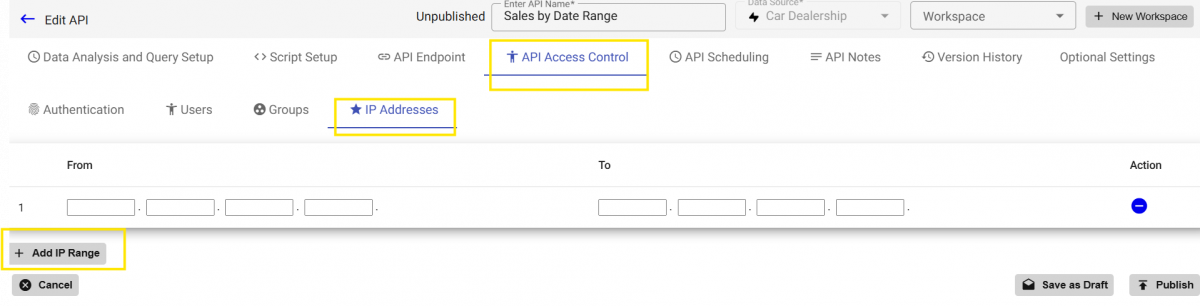